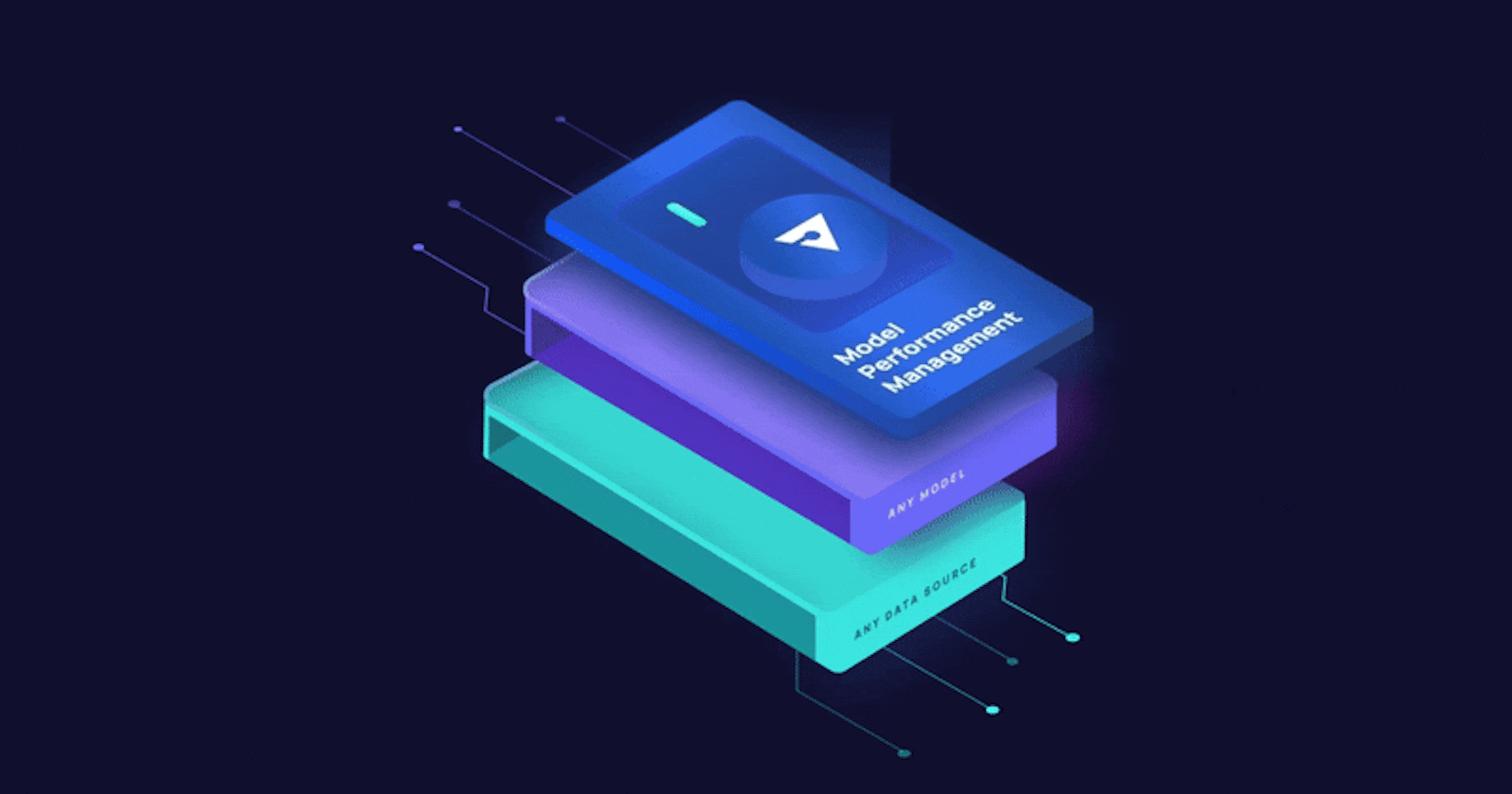
Building a Comprehensive Loan Underwriting Module
Streamlining Lending with Python Loan Underwriting
In the world of finance, loan underwriting is the backbone of responsible lending. It's the process that helps financial institutions assess the creditworthiness of applicants, making decisions about whether to approve or decline loan requests. Traditionally, this was a time-consuming and often subjective task. However, in the age of data and machine learning, automating loan underwriting has become not just a matter of efficiency but also a key to achieving unparalleled accuracy.
Imagine a bank or a fintech company dealing with hundreds or thousands of loan applications every day. Manually evaluating each applicant's credit history, income, and other factors is not only time-consuming but prone to human error. Automated loan underwriting using machine learning can help streamline the process, reduce the risk of errors, and ultimately, make better lending decisions.
In this comprehensive guide, we'll embark on a journey to build a loan underwriting module using Python. We'll cover the entire spectrum, from understanding the data through Exploratory Data Analysis (EDA) to preparing the data, training machine learning models, and making underwriting decisions. This guide is tailored for developers who have already built a loan application or a widget and are ready to take the next step in automating the underwriting process.
1. Exploratory Data Analysis (EDA)
Exploratory Data Analysis is the first step in understanding your dataset.
Univariate Analysis: This involves examining the distribution of each feature. In Python, you can create histograms using libraries like Matplotlib and Seaborn to visualize the distribution of numerical features.
pythonCopy codeimport matplotlib.pyplot as plt
import seaborn as sns
sns.histplot(df['age'], bins=20)
Bivariate Analysis: Next, explore how each feature relates to the target variable (e.g., loan approval status). Box plots and visualizations are useful for this purpose.
pythonCopy codesns.boxplot(x='loan_status', y='income', data=df)
Correlation Analysis: Checking for feature correlations is crucial to identify multicollinearity. Create a heatmap to visualize correlations between features.
pythonCopy codesns.heatmap(df.corr(), annot=True)
2. Data Preprocessing
Data preprocessing is essential to prepare your data for machine learning.
Handling Missing Values: Impute missing data using strategies like mean or median. The SimpleImputer
from Scikit-Learn helps with this.
pythonCopy codefrom sklearn.impute import SimpleImputer
imputer = SimpleImputer(strategy='mean')
X = imputer.fit_transform(X)
Handling Outliers: Address outliers as needed based on your EDA findings.
Categorical Encoding: Encode categorical variables using techniques like One-Hot Encoding with Scikit-Learn's OneHotEncoder
.
pythonCopy codefrom sklearn.preprocessing import OneHotEncoder
encoder = OneHotEncoder()
X_encoded = encoder.fit_transform(X_categorical)
Normalization/Standardization: Scale numerical features to ensure they are on a common scale. Scikit-Learn's StandardScaler
can help with this.
pythonCopy codefrom sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
3. Model Training & Tuning
Model training and tuning involve building and optimizing your machine learning model.
Hyperparameter Tuning: Search for the best hyperparameters using techniques like GridSearchCV. This helps optimize your model's performance.
pythonCopy codefrom sklearn.model_selection import GridSearchCV
parameters = {'C': [0.001, 0.01, 0.1, 1, 10], 'penalty': ['l1', 'l2']}
grid_search = GridSearchCV(LogisticRegression(), parameters)
grid_search.fit(X_train, y_train)
Cross-Validation: Implement k-fold cross-validation to rigorously assess your model's performance and ensure it generalizes well to unseen data.
Ensemble Methods: Consider employing ensemble methods like Random Forest or Gradient Boosting to improve your model's predictive power by combining multiple models.
4. Model Evaluation
Evaluate your model's performance using relevant metrics.
Performance Metrics: Assess your model's performance using metrics such as accuracy, precision, recall, and F1-score. The classification_report
function from Scikit-Learn is helpful for this purpose.
pythonCopy codefrom sklearn.metrics import classification_report
print("Classification Report:", classification_report(y_test, y_pred))
5. Prediction & Underwriting Decision
Finally, apply your trained model to make underwriting decisions for new loan applications.
Apply the Trained Model: Utilize the trained machine learning model to make underwriting decisions for new loan applications. Input applicant data, scale it using the StandardScaler (if applicable), and generate loan approval or rejection predictions.
Conclusion
Building a loan underwriting module is a multi-step process that requires a detailed understanding of the domain, as well as expertise in data science techniques. We've covered the essentials of EDA, data preprocessing, model tuning, and evaluation in this guide. Implementing these steps will put you on the path to creating a robust and reliable loan underwriting system.
Whether you're a data scientist aiming to break into the fintech industry or a seasoned professional looking to refine your skills, the techniques and tips outlined here will be invaluable in your journey to mastering loan underwriting.
That concludes our comprehensive guide on building a loan underwriting module! Feel free to adapt and extend the code and techniques to suit your specific requirements.